. Advertisement .
..3..
. Advertisement .
..4..
Along with CSS and HTM, the computer language, JavaScript, or JS, is one of the core technics of the World Wide Web. You can use Javascript for web development, game development, web applications, and many more. However, how to Show an Element if Checkbox is checked using JavaScript is a difficult problem in JavaScript. What can we do to show an element if Checkbox is checked? Read this article. We will give you some helpful information about this problem.
What Can We Do To Show an Element if Checkbox is checked using JavaScript?
Let’s follow these steps to show an element if Checkbox is checked using JavaScript:
- First, a
click
event listener must be added to the checkbox. - Check whether the checkbox is checked or not.
- If it is checked, let’s set the hidden element’s display property to block.
The HTML for the examples in this article is provided here. Look on it:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<title>Show an Element if Checkbox is checked using JavaScript</title>
</head>
<style>
#example_box {
display: none;
width: 300px;
height: 150px;
background-color: rgb(114, 250, 114);
color: rgb(15, 15, 15);
}
</style>
<body>
<p>Select an option:</p>
<div>
<input type="checkbox" id="exp_show" name="exp_show" />
<label for="exp_show"><b>Show the Element</b></label>
</div>
<div id="example_box"><i>The Element shown</i></div>
<script src="main.js"></script>
</body>
</html>
And below is the relevant JavaScript.
const checkbox = document.getElementById('exp_show');
const example_box = document.getElementById('example_box');
checkbox.addEventListener('click', function handleClick() {
if (checkbox.checked) {
example_box.style.display = 'block';
} else {
example_box.style.display = 'none';
}
});
Output:
......... ADVERTISEMENT .........
..8..
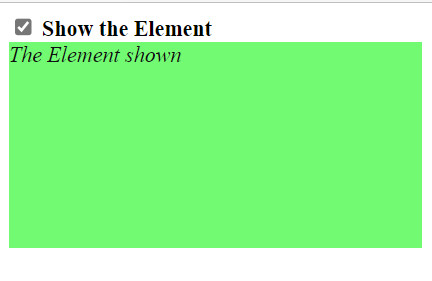
A click
event listener was added to the checkbox. The handleClick function is called each time the checkbox is clicked. If the checkbox is selected, you can set the div element’s display property to block to make it visible. The show property of the div is set to none, and the element is hidden if the checkbox is unchecked.
In the examples, we used the show property; however, depending on your use case, you could also need to use the visibility property. An element is deleted from the DOM and has no impact on the layout when its display attribute is set to none. The document is rendered as if it is absent.
As opposed to this, when an element’s visibility property is set to hidden, it still occupies space on the page but is hidden (not drawn). The element nevertheless influences your website as usual. Here is an illustration of displaying an element if a checkbox is selected using the visibility property.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<title>Show an Element if Checkbox is checked using JavaScript</title>
</head>
<style>
#example_box {
visibility: hidden;
width: 300px;
height: 150px;
background-color: rgb(114, 250, 114);
color: rgb(15, 15, 15);
}
</style>
<body>
<div>
<input type="checkbox" id="exp_show" name="exp_show" />
<label for="exp_show"><b>Show the Element</b></label>
</div>
<div id="example_box"><i>The Element shown</i></div>
<div>More content here</div>
<script src="main.js"></script>
</body>
</html>
And below is the relevant JavaScript.
const checkbox = document.getElementById('exp_show');
const example_box = document.getElementById('example_box');
checkbox.addEventListener('click', function handleClick() {
if (checkbox.checked) {
example_box.style.visibility = 'visible';
} else {
example_box.style.visibility = 'hidden';
}
});
Output:
......... ADVERTISEMENT .........
..8..
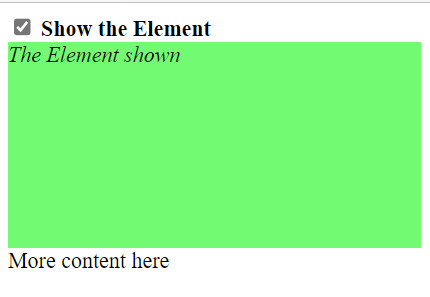
By loading the page, you can observe that the element occupies space even while it is hidden. The element impacts the page’s layout even when it is invisible as usual. If the element’s show attribute had been set to none, it would have been removed from the DOM and replaced on the page by the next element. It should be avoided whenever possible since changing the page’s content by using the display property to reveal or hide an element might be confusing.
The above solution is the best way for you to show an element if Checkbox is checked using JavaScript. Let’s apply it to get your desired results. If you want to learn more about “How To Check If A Checkbox Is Checked”, let’s keep reading this article. The following information is very helpful for you.
How To Check if Checkbox is checked
There are two states in a checkbox; they are checked and unchecked. You must do the following steps to determine a checkbox’s state: First, use a DOM technique like querySelector or getElementById() to select the checkbox (). Access the checkbox element’s checked attribute next. The checkbox is selected if the value of its checked property is true; otherwise, it is not. Look at the example below:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>JavaScript Checkbox</title>
</head>
<body>
<label for="accept">
<input type="checkbox" id="accept" name="accept" value="yes"> Accept
</label>
<script>
const cb = document.querySelector('#accept');
console.log(cb.checked); // false
</script>
</body>
</html>
In this example:
Make the HTML checkbox element first:
<label for="accept">
<input type="checkbox" id="accept" name="accept" value="yes"> Accept
</label>
Select the #accept checkbox, then check the checked property.
const cb = document.querySelector('#accept');
console.log(cb.checked);
You may see false
in the console because the checkbox is unchecked:
false
Conclusion
You can apply our methods when you have difficulty with the problem “How To Show an Element if Checkbox is checked using JavaScript“. These methods may seem complicated, but they will best solve your issue. Please fill in the blanks with questions and suggested responses to help us better assist you. Thank you for your reading!
Read more
Leave a comment